According Emil Eifrem the neo4j database outperforms relational backends with >1000x for many increasingly important use cases.
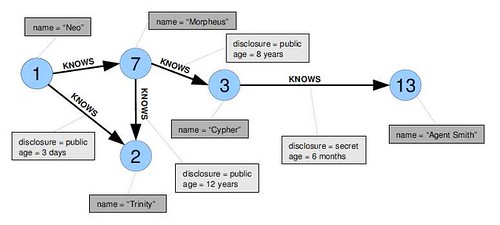
My intention was to make a place to discuss a different aspects of Java development and to review a Java frameworks, tools and interesting solutions.
InfoQueue has this excellent talk by Brian Goetz on the new features being added to Java SE 7 that will allow programmers to fully exploit our massively multi-processor future. While the talk is about Java it's really more general than that and there's a lot to learn here for everyone.
Brian starts with a short, coherent, and compelling explanation of why programmers can't expect to be saved by ever faster CPUs and why we must learn to exploit the strengths of multiple core computers to make our software go faster.
Some techniques for exploiting multiple cores are given in an equally short, coherent, and compelling explanation of why divide and conquer as the secret to multi-core bliss, fork-join, how the Java approach differs from map-reduce, and lots of other juicy topics.
The multi-core "problem" is only going to get worse. Tilera founder Anant Agarwal estimates by 2017 embedded processors could have 4,096 cores, server CPUs might have 512 cores and desktop chips could use 128 cores. Some disagree saying this is too optimistic, but Agarwal maintains the number of cores will double every 18 months.
An abstract of the talk follows though I would highly recommend watching the whole thing. Brian does a great job.
This implementation of chkconfig was inspired by the chkconfig command present in the IRIX operating system. Rather than maintaining configuration information outside of the /etc/rc[0-6].d hierarchy, however, this version directly manages the symlinks in /etc/rc[0-6].d. This leaves all of the configuration information regarding what services init
starts in a single location. chkconfig has five distinct functions: adding new services for
management, removing services from management, listing the current startup information for services, changing the startup information for services, and checking the startup state of a particular service. When chkconfig is run without any options, it displays usage
information. If only a service name is given, it checks to see if the service is configured to be started in the current runlevel. If it is, chkconfig returns true; otherwise it returns false. The
--level option may be used to have chkconfig query an alternative runlevel rather than the current one. If one of on, off, or reset is specified after the service name, chkconfig changes the startup information for the specified service. The on and off flags cause the service
to be started or stopped, respectively, in the runlevels being changed. The reset flag resets the startup information for the service to whatever is specified in the init script in question.
By default, the on and off options affect only runlevels 2, 3, 4, and 5, while reset affects all of the runlevels. The --level option may be used to specify which runlevels are affected.
Note that for every service, each runlevel has either a start script or a stop script. When switching runlevels, init will not re-start an already-started service, and will not re-stop a service that is not running.
For example, random.init has these three lines:
# chkconfig: 2345 20 80
# description: Saves and restores system entropy pool for \
# higher quality random number generation.
#startup script for Jakarta Tomcat
#
# chkconfig: 345 84 16
# description: Jakarta Tomcat Java Servlet/JSP Container
TOMCAT_HOME=/usr/local/bin/apache-tomcat-6.0.16
TOMCAT_START=/usr/local/bin/apache-tomcat-6.0.16/bin/startup.sh
TOMCAT_STOP=/usr/local/bin/apache-tomcat-6.0.16/bin/shutdown.sh
TOMCAT_RUN=/usr/local/bin/apache-tomcat-6.0.16/bin/catalina.sh
#Necessary environment variables
export CATALINA_HOME=/usr/local/bin/apache-tomcat-6.0.16
# Source function library.
. /etc/rc.d/init.d/functions
# Source networking configuration.
. /etc/sysconfig/network
# Check that networking is up.
[ ${NETWORKING} = "no" ] && exit 0
#Check for tomcat script
if [ ! -f $TOMCAT_HOME/bin/catalina.sh ]
then
echo "Tomcat not available..."
exit
fi
start() {
echo -n "Starting Tomcat: "
su - root -c $TOMCAT_START
echo
touch /var/lock/subsys/tomcatd
# We may need to sleep here so it will be up for apache
# sleep 5
#Instead should check to see if apache is up by looking for http.pid
}
run() {
echo -n "Starting Tomcat: "
su - root -c $TOMCAT_START
echo
touch /var/lock/subsys/tomcatd
# We may need to sleep here so it will be up for apache
# sleep 5
#Instead should check to see if apache is up by looking for http.pid
}
stop() {
echo -n $"Shutting down Tomcat: "
su - root -c $TOMCAT_STOP
rm -f /var/lock/subsys/tomcatd.pid
echo
}
status() {
ps ax --width=1000 | grep "[o]rg.apache.catalina.startup.Bootstrap
start" | awk '{printf $1 " "}' | wc | awk '{print $2}' > /tmp/tomcat_process_count.txt
read line < /tmp/tomcat_process_count.txt if [ $line -gt 0 ]; then echo -n "tomcatd ( pid " ps ax --width=1000 | grep "[o]rg.apache.catalina.startup.Bootstrap start" | awk '{printf $1 " "}' echo -n ") is running..." else echo -n "Tomcat is stopped" fi } case "$1" in start) start ;; run) run ;; stop) stop ;; restart) stop sleep 3 start ;; status) status ;; *) echo "Usage: tomcatd {start|stop|restart|status}" exit 1 esac
rpm -Uvh http://download.fedora.redhat.com/pub/epel/5/i386/epel-release-5-3.noarch.rpm
yum install ssmtp
root=noreply@youdomain.com
AuthUser=noreply@yourdomain.com
AuthPass=password
FromLineOverride=YES
mailhub=smtp.gmail.com:587
UseSTARTTLS=YES
echo "test" | ssmtp -v -s "Test" genadyg@exelate.com
ssmtp myemailaddress@gmail.com < msg.txt
To: toaddress@gmail.com
From: fromaddress@gmail.com
Subject: alert
Message text
Went Walkabout. Brought back Google Wave Google Wave Drips With Ambition. A New Communication Platform For A New Web
Google Wave is a new communication and collaboration platform based on hosted XML documents (called waves) supporting concurrent modifications and low-latency updates. This platform enables people to communicate and work together in new, convenient and effective ways. We will offer these benefits to users of Google Wave and we also want to share them with everyone else by making waves an open platform that everybody can share. We welcome others to run wave servers and become wave providers, for themselves or as services for their users, and to "federate" waves, that is, to share waves with each other and with Google Wave. In this way users from different wave providers can communicate and collaborate using shared waves. We are introducing the Google Wave Federation Protocol for federating waves between wave providers on the Internet.
Here are the initial white papers that are available to complement the Google Wave Federation Protocol:
The Google Wave APIs are documented here.